In this post, I’ll show you how to create a new user, modify an existing user and remove an old user in Windows Active Directory using PowerShell.
Create a Single User in Active Directory
The PowerShell New-ADUser CMDlet is use for creating a user in Active Directory.
New-ADUser doesn’t have many mandatory parameters but you can use different parameters while creating a new user.
New-ADUser –SamAccountName “username” –DisplayName “username” –givenName “Username” –Surname “surname” –AccountPassword (ReadHost –AsSecureString “Message”) –Enabled $true –Path ‘CN=Users,DC=Doc,DC=Com’ –CannotChangePassword $false –ChangePasswordAtLogon $true –PasswordNeverExpires $false -EmailAddress “email” –EmployeeID “ID” –Department “string”
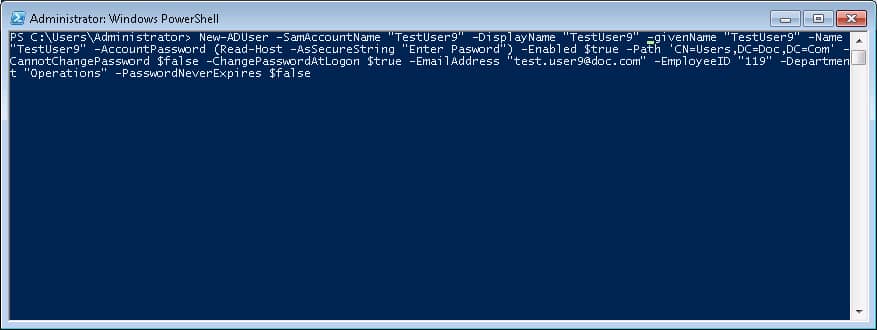
Below are the descriptions of parameters used in the above CMDlet
After executing the command, the PowerShell will ask for the password.
Enter the password and the user will be created.
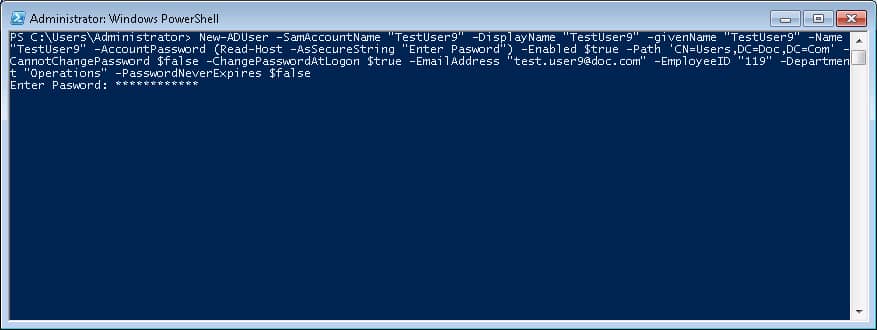
Creating Bulk Users in Active Directory
It’s required to create a CSV file before going to create the bulk users through PowerShell using the Import-CSV CMDlet. Following is a screenshot of the required CSV file.
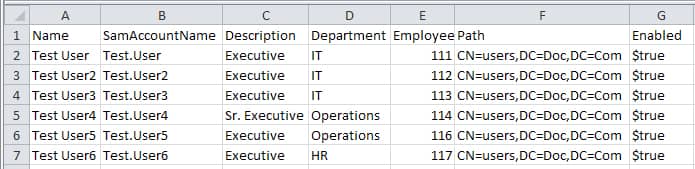
Now, execute the following command to create bulk users in AD.
Import-CSV d:\Share\testing.csv | New-ADUser
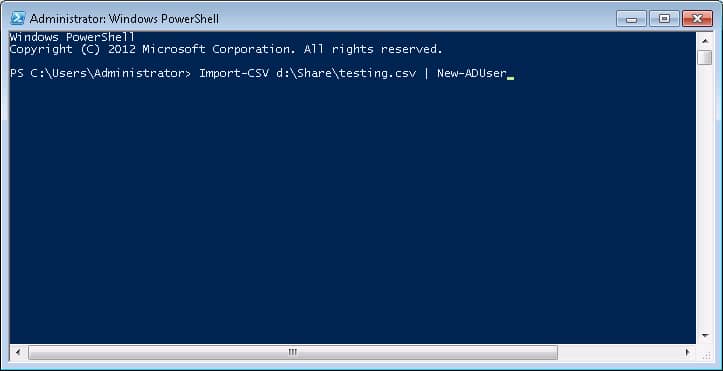
The Import-CSV provides pipeline input to the New-ADUser CMDlet. It processes the values of the CSV file to create the new users. Executing this command will load the Active Directory module first.
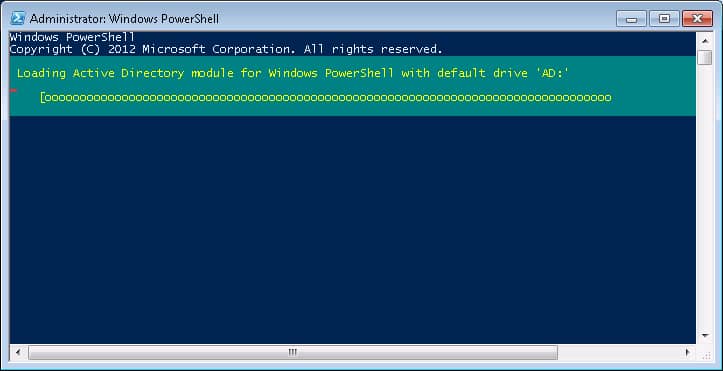
After completing the action, you’ll return to the same prompt.
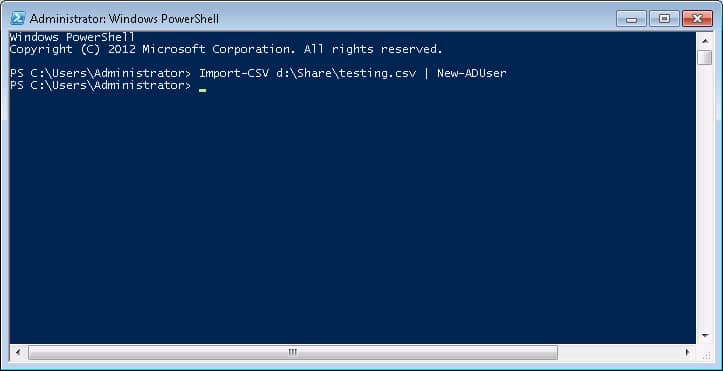
Check the Active Directory for the newly created users.
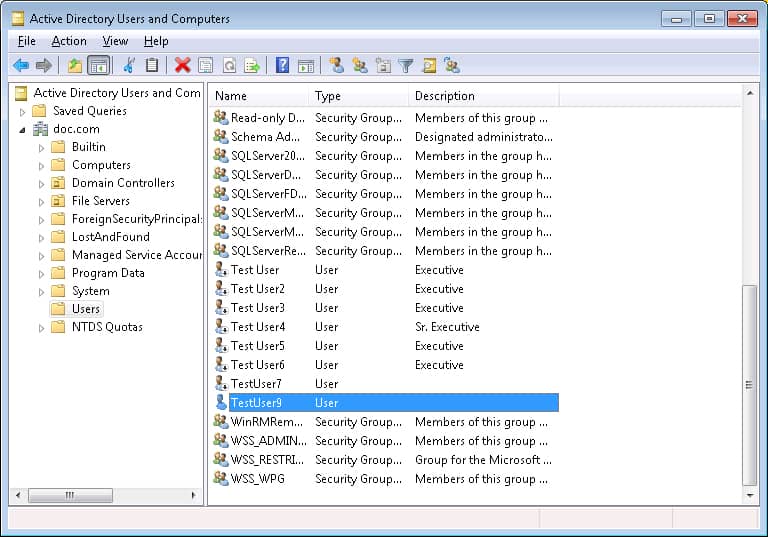
Modify Users in Active Directory
Use the Set-ADUser CMDlet to modify the user in AD.
Set-ADUser –Identity “CN=TestUser7,CN=Users,DC=www,DC=DOC,DC=com” –SamAccountName “TestUser7” –LogonWorkStations “Test”
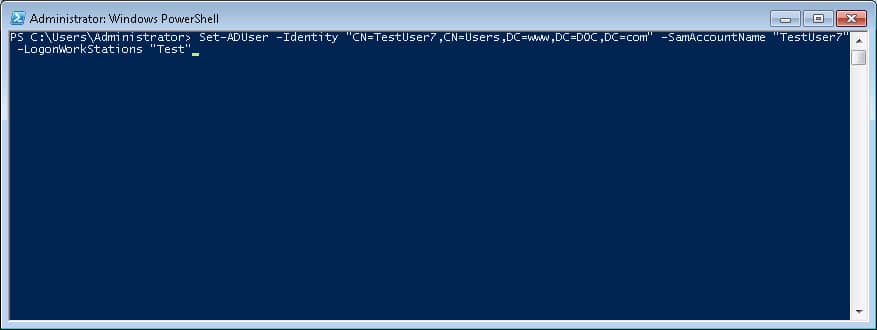
Some of the available parameters for this CMDlet are listed below.
Reset Password for AD Users
You can reset the password of a user with Set-ADAccountPassword CMDlet.
Set-ADAccountPassword –Identity “CN=TestUser7,CN=Users,DC=www,DC=DOC,DC=com” –SamAccountName “TestUser7” –LogonWorkStations “Test”
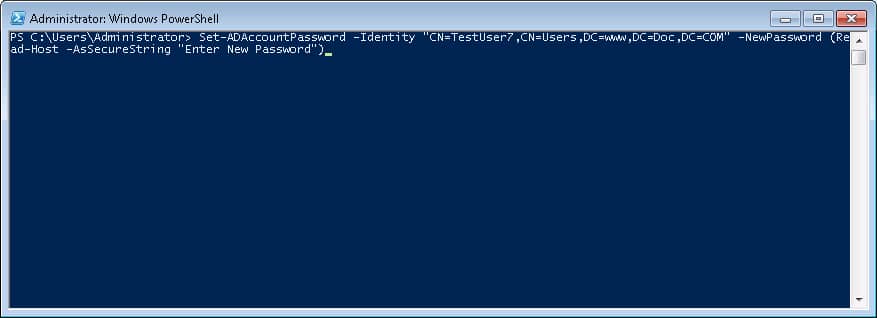
Some of the acceptable parameters for this CMDlet are listed below.
Removing a Active Directory User Account
You can remove a user account using the Remove-ADUser CMDlet.
Remove-ADUser –Identity “CN=Username,CN=Users,DC=doc,DC=com”
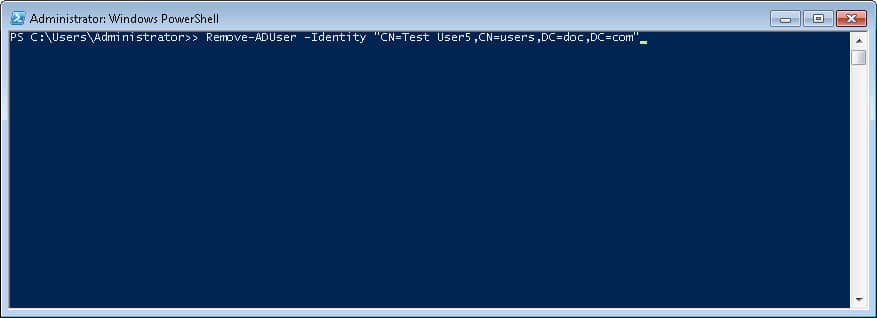
Pressing the Enter key will ask for confirmation to delete the user.
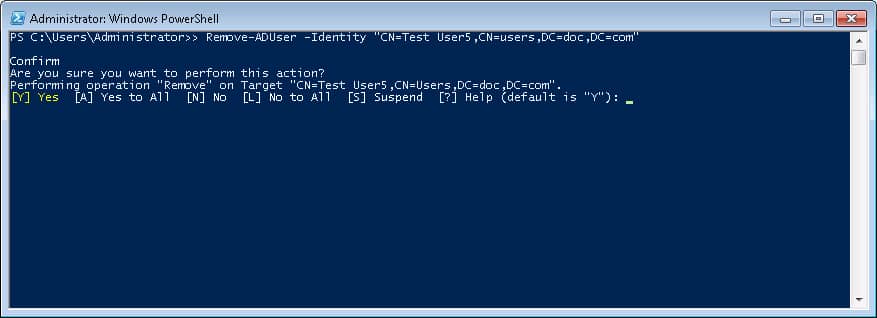
Press Y to confirm the action.
Conclusion
To ensure security of your Active Directory it is important to keep track of user creation, modification and deletion activities. With native methods, it is very difficult to monitor these activities. Lepide Active Directory Auditor can help you to audit user creation, modification and deletion in real time with other important AD changes.